Excel Ray Tracing with Help from C
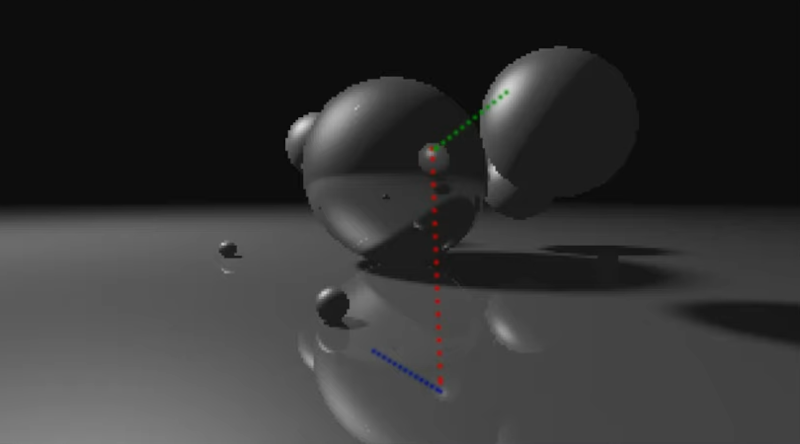
It isn’t news that [s0lly] likes to do ray tracing using Microsoft Excel. However, he recently updated his set up to use functions in a C XLL — a DLL, …read more Continue reading Excel Ray Tracing with Help from C
Collaborate Disseminate
It isn’t news that [s0lly] likes to do ray tracing using Microsoft Excel. However, he recently updated his set up to use functions in a C XLL — a DLL, …read more Continue reading Excel Ray Tracing with Help from C
Geeks of a certain vintage will have fond memories of games that were simplistic by today’s standards, but drew one in all the same. Their low fidelity graphics were often …read more Continue reading Custom Compression Squeezes Classic Computer Choruses Into A Tiny Controller
Computing is really all about order. If you can take data, apply an operation to it, and get the same result every single time, then you have a stable and reliable computing system.
So it makes total sense that there is Operator Precedence. This is also called Order of Operations, and it dictates which computations will be performed first, and which will be performed last. To get the same results every time, you must perform addition, multiplication, power functions, bitwise math, and all other calculations in a codified order.
The question I’ve had on my mind lately is, does this …read more
Continue reading Ask Hackaday: Is There a Legit Use for Operator Precedence?
Let’s be honest, no one likes to see their program crash. It’s a clear sign that something is wrong with our code, and that’s a truth we don’t like to see. We try our best to avoid such a situation, and we’ve seen how compiler warnings and other static code analysis tools can help us to detect and prevent possible flaws in our code, which could otherwise lead to its demise. But what if I told you that crashing your program is actually a great way to improve its overall quality? Now, this obviously sounds a bit counterintuitive, after all …read more
Continue reading Crash your code – Lessons Learned From Debugging Things That Should Never Happen™
A little while back, we were talking about utilizing compiler warnings as first step to make our C code less error-prone and increase its general stability and quality. We know now that the C compiler itself can help us here, but we also saw that there’s a limit to it. While it warns us about the most obvious mistakes and suspicious code constructs, it will leave us hanging when things get a bit more complex.
But once again, that doesn’t mean compiler warnings are useless, we simply need to see them for what they are: a first step. So today …read more
Continue reading Warnings On Steroids – Static Code Analysis Tools
Dividing by zero — the fundamental no-can-do of arithmetic. It is somewhat surrounded by mystery, and is a constant source for internet humor, whether it involves exploding microcontrollers, the collapse of the universe, or crashing your own world by having Siri tell you that you have no friends.
It’s also one of the few things gcc
will warn you about by default, which caused a rather vivid discussion with interesting insights when I recently wrote about compiler warnings. And if you’re running a modern operating system, it might even send you a signal that something’s gone wrong and let you …read more
Continue reading Creating Black Holes: Division By Zero In Practice
If there’s one thing C is known and (in)famous for, it’s the ease of shooting yourself in the foot with it. And there’s indeed no denying that the freedom C offers comes with the price of making it our own responsibility to tame and keep the language under control. On the bright side, since the language’s flaws are so well known, we have a wide selection of tools available that help us to eliminate the most common problems and blunders that could come back to bite us further down the road. The catch is, we have to really want it …read more
Continue reading Warnings Are Your Friend – A Code Quality Primer
Shared libraries are our best friends to extend the functionality of C programs without reinventing the wheel. They offer a collection of exported functions, variables, and other symbols that we can use inside our own program as if the content of the shared library was a direct part of our code. The usual way to use such libraries is to simply link against them at compile time, and let the linker resolve all external symbols and make sure everything is in place when creating our executable file. Whenever we then run our executable, the loader, a part of the operating …read more
Continue reading It’s All In The Libs – Building A Plugin System Using Dynamic Loading
In the first part of this series, we covered the basics of pointers in C, and went on to more complex arrangements and pointer arithmetic in the second part. Both times, we focused solely on pointers representing data in memory.
But data isn’t the only thing residing in memory. All the program code is accessible through either the RAM or some other executable type of memory, giving each function a specific address inside that memory as entry point. Once again, pointers are simply memory addresses, and to fully utilize this similarity, C provides the concept of function pointers. Function pointers …read more
Continue reading Directly Executing Chunks of Memory: Function Pointers In C
In our first part on pointers, we covered the basics and common pitfalls of pointers in C. If we had to break it down into one sentence, the main principle of pointers is that they are simply data types storing a memory address, and as long as we make sure that we have enough memory allocated at that address, everything is going to be fine.
In this second part, we are going to continue with some more advanced pointer topics, including pointer arithmetic, pointers with another pointer as underlying data type, and the relationship between arrays and pointers. But first, …read more
Continue reading When 4 + 1 Equals 8: An Advanced Take On Pointers In C